How to Easily Add a Contact Form to Your HTML/CSS Static Portfolio Website
In this post, I'll walk you through the steps I took to integrate Web3Forms into my HTML/CSS portfolio.
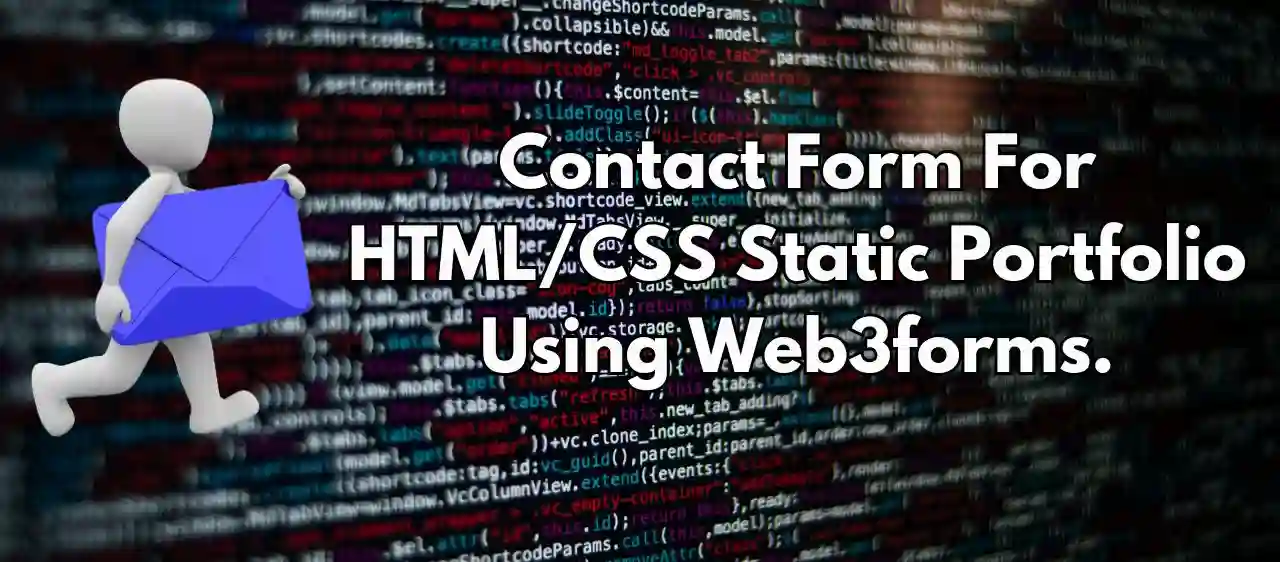
Creating a contact form for my personal blog without a backend can be challenging. However, by using the Web3Forms API, I could easily set up a functional and customizable contact form. In this post, I’ll walk you through the steps I took to integrate Web3Forms into my HTML/CSS portfolio.
Why Web3Forms?
I chose Web3Forms because it’s a free service that allows you to receive HTML contact form submissions directly in your email inbox without any server or backend code. It’s customizable, easy to integrate, and perfect for modern websites.
What is Web3Forms?
Web3Forms is a service that provides contact forms for modern websites. It lets you receive form submissions directly in your email inbox using their contact form API service. This makes it an excellent choice for static websites that lack backend support.
Setting Up the HTML Structure
Here is the HTML structure I used for my contact form:
<div class="contactform">
<form action="https://api.web3forms.com/submit" method="POST">
<!-- Replace with your Access Key -->
<input type="hidden" name="access_key" value="Replace with your Access Key">
<input type="hidden" name="subject" value="New Contact Form Submission From Portfolio">
<input type="hidden" name="redirect" value="https://">
<!-- Form Inputs. Each input must have a name="" attribute -->
<input type="text" name="name" placeholder="Name" required>
<input type="email" name="email" placeholder="Email" required>
<textarea name="message" placeholder="Message" required></textarea>
<!-- Honeypot Spam Protection -->
<input type="checkbox" name="botcheck" class="hidden" style="display: none;">
<button type="submit">Submit Form</button>
<p id="result"></p>
</form>
</div>
Styling with CSS
I styled the form based on my design preferences. Here is an example of how I styled it:
.contactform {
width: 100%;
max-width: 600px;
margin: 0 auto;
}
.contactform input,
.contactform textarea {
width: 100%;
padding: 10px;
margin: 10px 0;
}
.contactform button {
padding: 10px 20px;
background-color: #333;
color: #fff;
border: none;
cursor: pointer;
}
.contactform button:hover {
background-color: #555;
}
Integrating Web3Forms API
To integrate Web3Forms API, I included the API endpoint and my access key in the form’s action
attribute. Additionally, I needed to customize the redirect URL to point to my own thank-you page instead of the default one provided by Web3Forms. Here is the line of code that handles the redirection:
<input type="hidden" name="redirect" value="Redirect page URL">
JavaScript for Form Submission
I used JavaScript to handle form submission and display a message to the user. Here is the code:
const form = document.getElementById('form');
const result = document.getElementById('result');
form.addEventListener('submit', function(e) {
e.preventDefault();
const formData = new FormData(form);
const object = Object.fromEntries(formData);
const json = JSON.stringify(object);
result.innerHTML = "Please wait..."
fetch('https://api.web3forms.com/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json'
},
body: json
})
.then(async (response) => {
let json = await response.json();
if (response.status == 200) {
result.innerHTML = "Form submitted successfully";
} else {
console.log(response);
result.innerHTML = json.message;
}
})
.catch(error => {
console.log(error);
result.innerHTML = "Something went wrong!";
})
.finally(function() {
form.reset();
setTimeout(() => {
result.style.display = "none";
}, 3000);
});
});
// Clear form after submission
window.onload = function() {
// Reset the form fields when the page loads
document.getElementById("form").reset();
};
Conclusion
Using Web3Forms was a straightforward solution for adding a contact form to my HTML/CSS portfolio. It’s free, safe, and easy to integrate, making it an excellent choice for anyone who needs a functional contact form without a backend. I highly recommend Web3Forms for anyone looking for a simple and effective contact form solution.